I had over 1,000 audio files in the .mpga
format and I wanted to convert them all to .mp3
format. For this, I wrote a Python script that does the conversion with style.
I put all the code inside this GitHub repository.
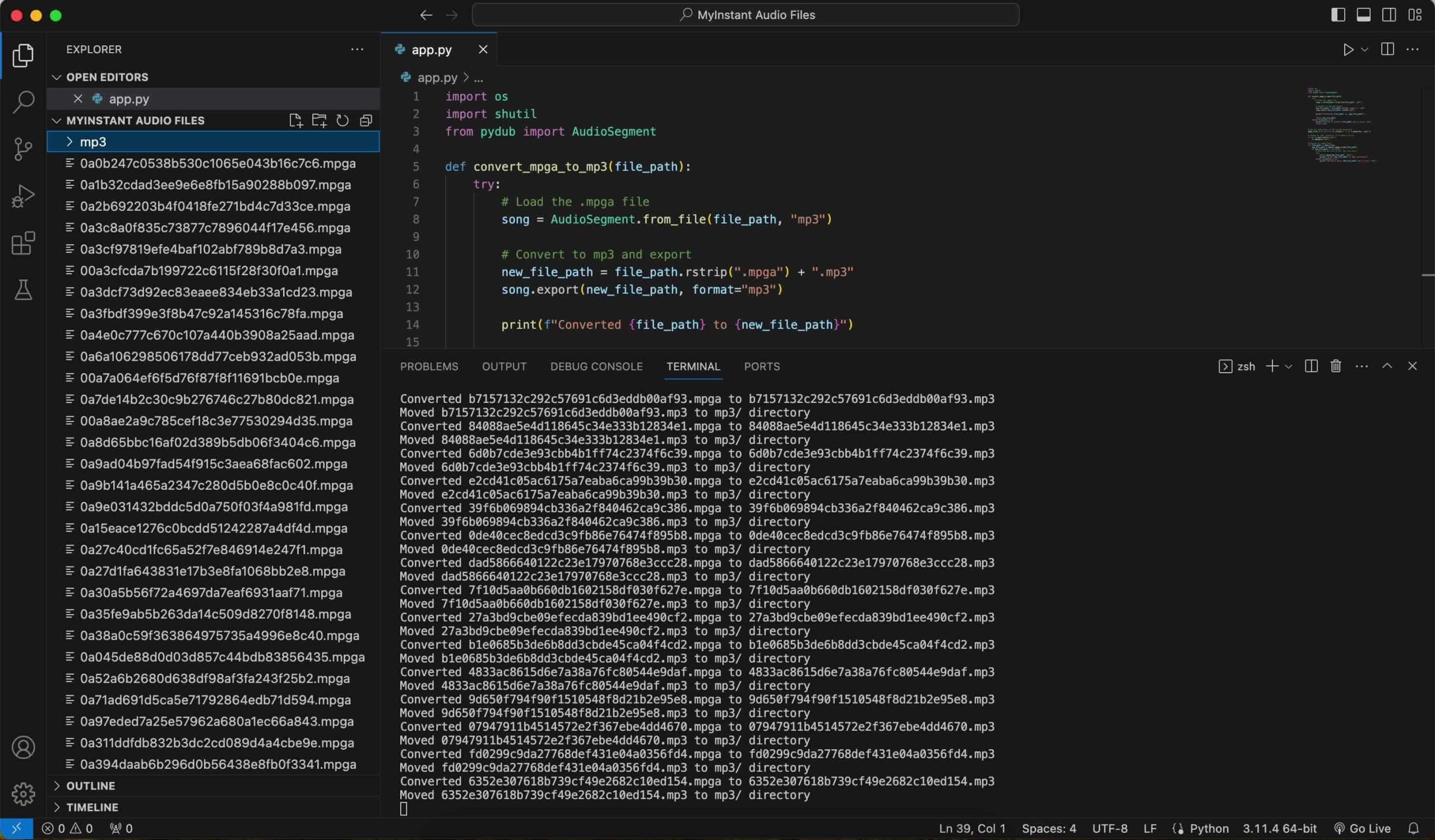
Now, let’s look at how I converted those 1,000+ files.
1. Put all the .mpga
files in a folder
Put all your audio files in a folder and open the VS Code or any other code editor you choose.
2. Create app.py in the same folder
Create the app.py file in the same folder and copy-paste the following code into the file.
import os
import shutil
from pydub import AudioSegment
def convert_mpga_to_mp3(file_path):
try:
# Load the .mpga file
song = AudioSegment.from_file(file_path, "mp3")
# Convert to mp3 and export
new_file_path = file_path.rstrip(".mpga") + ".mp3"
song.export(new_file_path, format="mp3")
print(f"Converted {file_path} to {new_file_path}")
return new_file_path
except Exception as e:
print(f"Failed to convert {file_path} due to error: {e}")
return None
# Get all mpga files in the current directory
mpga_files = [f for f in os.listdir('.') if f.endswith('.mpga')]
# Create an 'mp3' directory if it doesn't exist
if not os.path.exists('mp3'):
os.makedirs('mp3')
# Convert all mpga files
for file_path in mpga_files:
new_file_path = convert_mpga_to_mp3(file_path)
if new_file_path is not None:
# Move the mp3 file to the 'mp3' directory
try:
shutil.move(new_file_path, 'mp3/')
print(f"Moved {new_file_path} to mp3/ directory")
except Exception as e:
print(f"Failed to move {new_file_path} due to error: {e}")
3. Run the Python code
Install the pydub
library by running the pip install pydub
command in the terminal, and now run the Python code by running the following command in the terminal:
python app.py
And as soon as the code runs, converted .mp3
files will start getting saved inside a folder /mp3
in the main folder.
Note that you may need to use pip3 and python3 instead of pip and python, depending upon the Python version and setup your computer has.
That’s it! You can go through this GitHub repo to learn more.
Hope this helps.
Leave a Reply